Input Output in Prolog Part 1 until 5
This is our next Tutorial. This tutorial consist of five Tutorial about Input and Output in Prolog.
First, We'll show about making lower case in Prolog from User's Input. This is the source code :
/* Source Code Lower Case */
lowercase:-get0(A),process(A).
process(13).
process(A):-A=\=13,converter(A,B),put(B),makelower.
converter(A,B):-A>=65,A=<90,b>90.
This is mean if user type "lowercase", Program will ready to receive Input from user. The function of command get0() is to change one or more character to be ASCII Value. Then the ASCII value read by command process. After then, process initialize ASCII value 13 (13 means marriage return or Enter Key). If user type Enter Key, program will check the ASCII value which handled by command process before. If command proccess don't read an "Enter Key" again, Program start function converter which have function to convert ASCII value from 65 (65 is ASCII value of Upper Case A) until 90 (90 is ASCII value of Upper Case Z) by sum it by 32 (Because lower case of A is 97 in ASCII. It's same with another alphabet until Z, just sum it by 32). Then, if in process of convert, program read another character (not alphabet) Program just print it without sum the ASCII value by 32.
Step 1
Type the source code in text editor (ex. notepad).
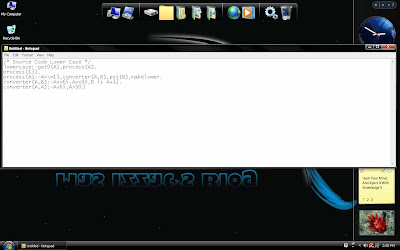
(Click the image if you can't see well)
Step 2
Save it at directory My Documents\Prolog and named it by extention (*.pl) or (*.pro). For example named it "lowercase.pro".
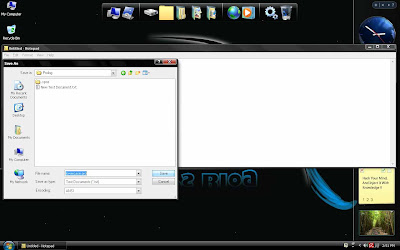
(Click the image if you can't see well)
Step 3
Open it with command consult.
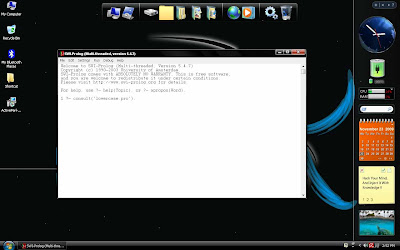
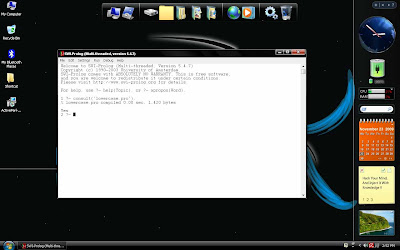
(Click the image if you can't see well)
Step 4
Now, let's check our program. Type "lowercase" (without quotes) and hit Enter.
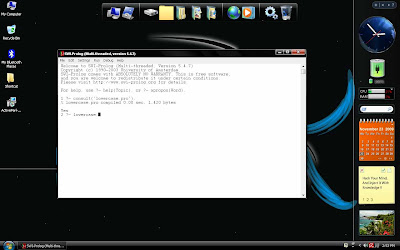
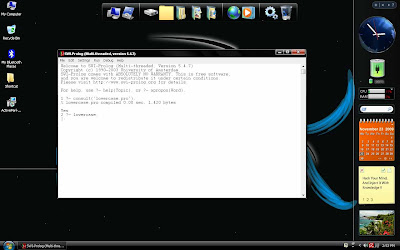
(Click the image if you can't see well)
Step 5
Try to type Anything character. For example type like this : "This is an Example 123 inCLUDing numbers and symbols +-*/@[] XYz" (Without quotes) and hit Enter
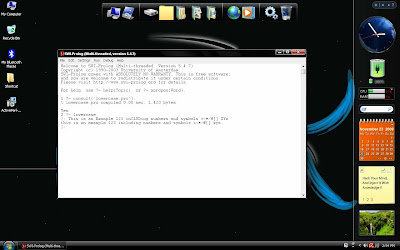
(Click the image if you can't see well)
Step 6
Let's try with different input : "ThIs IS jUSt WoRD tO Try ThE PRoGRAm !@#$%^&*()_+" (remember without quotes and hit Enter after type it)
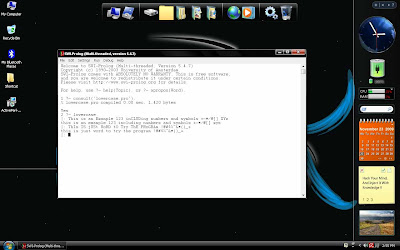
(Click the image if you can't see well)
This is the second Tutorial. Now, We will show about how to make a program in Prolog which can copy character from a file to another file.
First, let's make a logic from the program. The logic is the program must open the file and check the line per line. In each line, the program must know about the end line and then check the next line. In Prolog, There is command to Open and Write to another file. The commands are see and tell. Another commands are seeing and telling. Seeing and telling must be a variable and will normally be unbound. So, We can write the code like this :
/* Source Code Input from File */
copyterms(Infile,Outfile):-seeing(S),telling(T),see(Infile),tell(Outfile),copy_characters,seen,see(S),told,tell(T).
copy_characters:-read(N),process(N).
process(end_of_file).
process(N):-N\=end_of_file,write(N),write('.'),nl,copy_characters.
This code means that predicate copyterms must be input by file input and file output. Seeing and see in this source code have variable for input, the variable is S. Telling and tell have variable T. The precicate copy_character will read each character and the result of it will be handled by predicate process. Predicate process initialize String "end_of_file". And then, the program will check each character which handled by predicate process. If the character not a String "end_of_file", the program will print it.
Step 1
Type the source code to the text editor (Ex. Notepad)
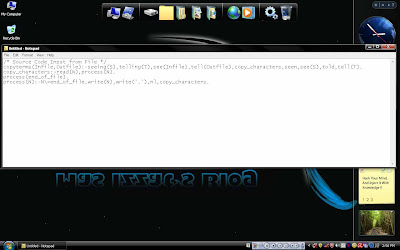
(Click the image if you can't see well)
Step 2
Save it at directory My Documents\Prolog. Save it by extention (*.pl) or (*.pro). For example named it "copyterms.pro".
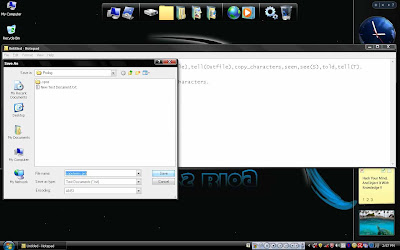
(Click the image if you can't see well)
Step 3
Make two files (Recommended by extention *.txt) at directory My Documents\Prolog. You must make 2 files. One for Input, and the another to output. For example : named it "input.txt" and "output.txt".
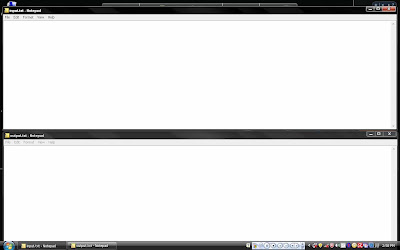
(Click the image if you can't see well)
Step 4
Start Prolog and open the source code with command consult.
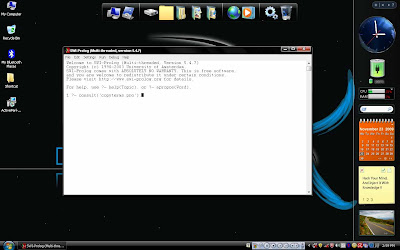
(Click the image if you can't see well)
Step 5
Now, open the input.txt and type like this :
'first term'. 'second term'.
'third term'.
fourth. 'fifth term'.
sixth.
And then save it.
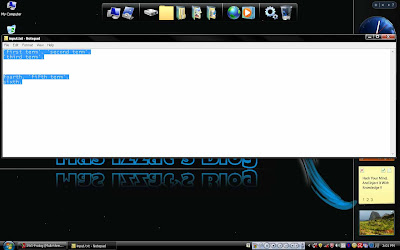
(Click the image if you can't see well)
Step 6
Type like this on Prolog : copyterms('input.txt','output.txt').
And hit Enter.
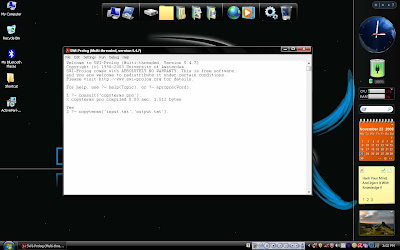
(Click the image if you can't see well)
Step 7
If Prolog show the notification "Yes", So succesfully copy the character. Now, check your output file and see the characters have been copied successfully.
Note : If your file have any blank line, the program will delete it.
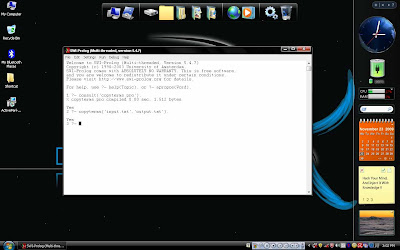
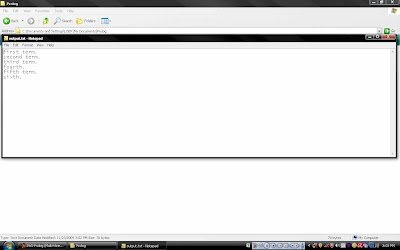
(Click the image if you can't see well)
Step 8
Let's try with different input. Type like this in your input file :
'I am'. just.
check. 'my program'.
'I hope'. this.
is.
can. compiled.
successfully.
And Save it.
Note : You must type '' mark if your input in more than one word. For example : 'I am'. And your each input must ended by stop mark ".".
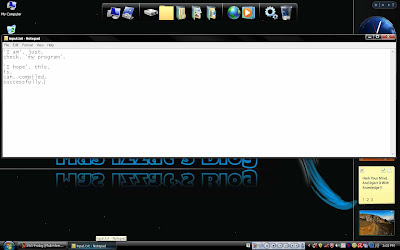
(Click the image if you can't see well)
Step 9
Type like this on Prolog again : copyterms('input.txt','output.txt'). And hit Enter.
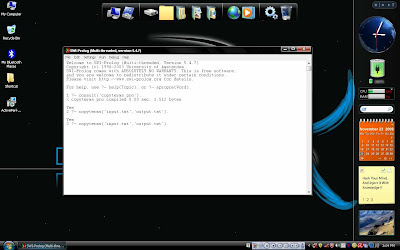
(Click the image if you can't see well)
Step 10
If Prolog show the notification "Yes", So succesfully copy the character. Now, check your output file and see the characters have been copied successfully.
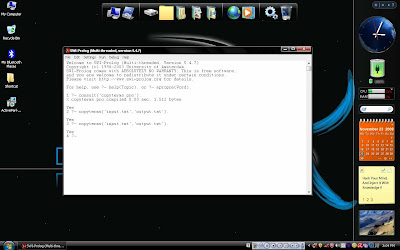
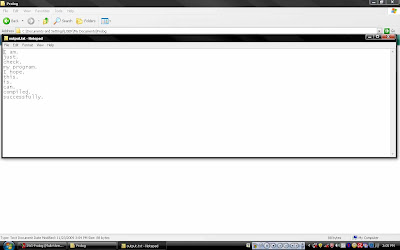
(Click the image if you can't see well)
This is the third tutorial from us. We will show about how to read one by one character from a file and convert it to ASCII value on Prolog.
Like usually, let's make the logic of program.
First, program will open a file. And then program will read one by one character. After then program will convert the character to ASCII value and the last print it on screen.
We can write the logic like this :
/* Source Code */
readfile(O):-seeing(S),see(O),readeachcharacter,readeachcharacter,readeachcharacter,readeachcharacter,readeachcharacter,readeachcharacter,readeachcharacter,readeachcharacter,readeachcharacter,readeachcharacter,seen,see(S).
readeachcharacter:-get0(A),write(A),nl.
This source code means that predicate readfile must be input by the input file name. After that program will open the file with predicate see. The predicate readeachcharacter use to convert one by one character which read by predicate readfile to be ASCII value (By predicate get0). Predicate readeachcharacter use to convert A CHARACTER from file, so We must type the predicate readeachcharacter same with the total of character will be converted.
For example, We want to convert ten character from file, So We must type predicate readeachcharacter ten times.
Step 1
Type the logic to text editor (ex. Notepad)
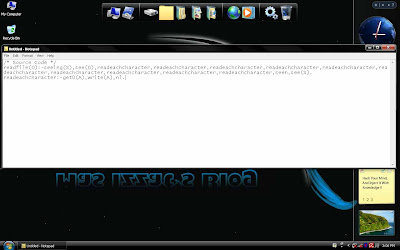
(Click the image if you can't see well)
Step 2
Save it at directory My Documents\Prolog. Save it by extention (*.pl) or (*.pro). For example named it "readfile.pro".
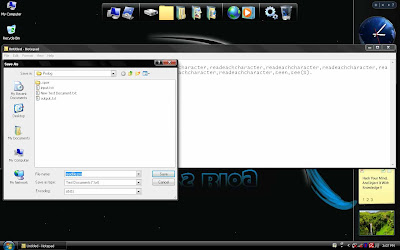
(Click the image if you can't see well)
Step 3
Make a file (Recommended by extention *.txt) at directory My Documents\Prolog. For example : named it "testa.txt".
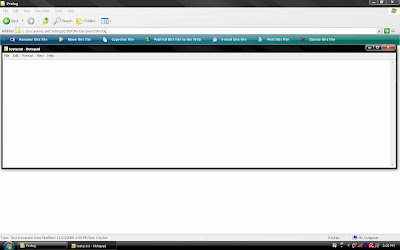.jpg)
(Click the image if you can't see well)
Step 4
Start Prolog and open the source code with command consult.
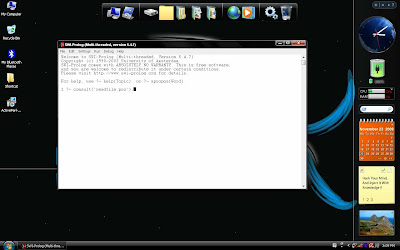
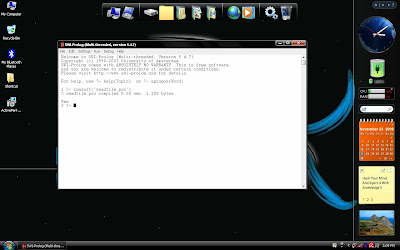
(Click the image if you can't see well)
Step 5
Now, open the input.txt and type like this :
abcde
fghij
And then save it.
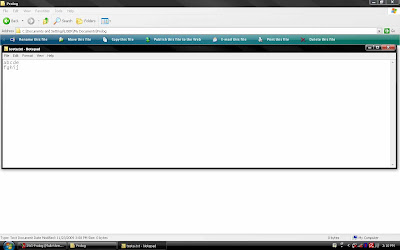
(Click the image if you can't see well)
Step 6
Type like this on Prolog : readfile('testa.txt').
And hit Enter.
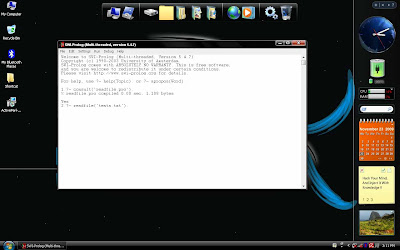
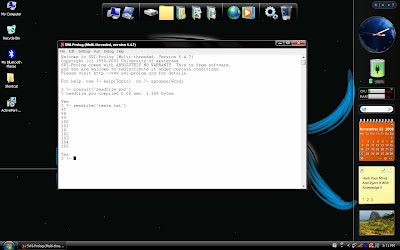
(Click the image if you can't see well)
Step 7
You can try with different word. But don't forget to change the total predicate readeachfile if you want to convert more or less character.
This is the fourth tutorial. In this tutorial, We will show about how to combine a file with another using Prolog.
Now, let's make the logic of program.
First, Program will open the first file. Then read each character in it. And then write the same charater in the output file.
Secondly, Program will open the the second file. Then read each character in it. After then write the same character in the output file.
So, We can type like this :
/* Source Code from The Logic */
combine(File1,File2,Out):-seeing(S),telling(T),tell(Out),see(File1),copycharacter,seen,see(File2),copycharacter,seen,see(S),nl,told,telling(T).
copycharacter:-read(N),process(N).
process(end).
process(N):-N\=end,write(N),nl,copycharacter.
This source code means that predicate combine must be input by name of the first file, the second file and the output file. After predicate combine receive the name of files, it will open the first file, read each character and write the same character in the output file. And then open the second file, read each character and write in the output file. On process, if character not identic with String "end", Program will copy it to the output file.
Step 1
Type the logic to text editor (ex. Notepad)
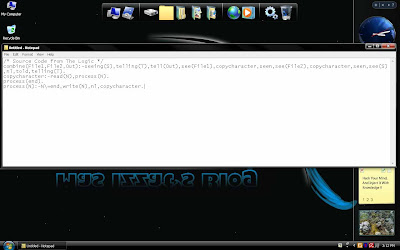
(Click the image if you can't see well)
Step 2
Save it at directory My Documents\Prolog. Save it by extention (*.pl) or (*.pro). For example named it "combine.pro".
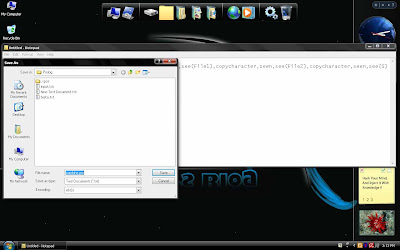
(Click the image if you can't see well)
Step 3
Make three files (Recommended by extention *.txt) at directory My Documents\Prolog. For example : named it "firstfile.txt", "secondfile.txt" and "output.txt".
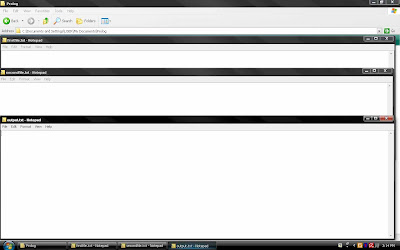
(Click the image if you can't see well)
Step 4
Start Prolog and open the source code with command consult.
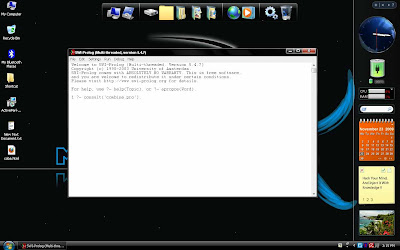
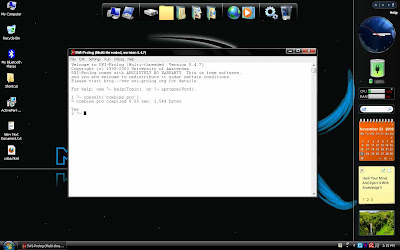
(Click the image if you can't see well)
Step 5
Now, open the firstfile.txt and type like this :
just.
try.
the.
program.
end.
And Save it.
Note : Don't forget to type the dot mark "." at every last word and must ended by type "end".
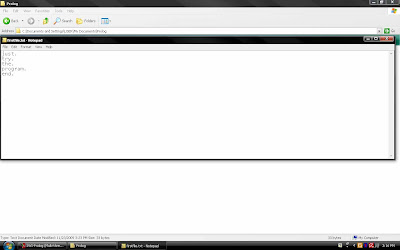
(Click the image if you can't see well)
Step 6
And then open the secondfile.txt and type like this :
but.
do.
not.
forget.
to.
type.
the.
dot.
mark.
and.
must.
ended.
by.
string-end.
end.
And Save it.
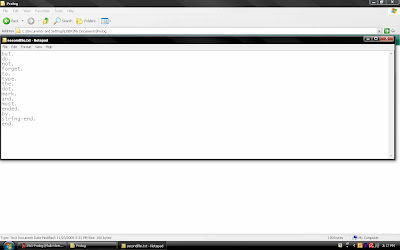
(Click the image if you can't see well)
Step 7
Now, type like this on Prolog :
combine('firstfile.txt','secondfile.txt','output.txt').
And hit Enter
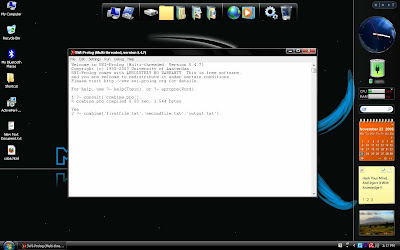
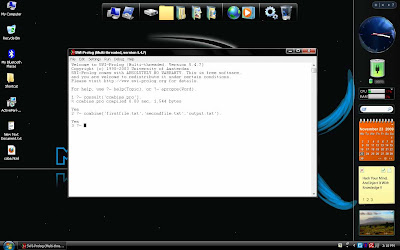
(Click the image if you can't see well)
Step 8
Open the output file to check the character.
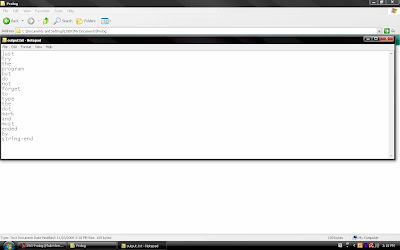
(Click the image if you can't see well)
Step 9
You can try the different word by yourself.
This the last tutorial from us now. This tutorial is about how to read each character from 2 files and check the character is the same character or not.
Okay, let's make the logic.
First, program will open the first file and the second file and then read the each character.
Secondly, check the character from first file and second file. If the character from the first file and the second file is identic, the program will print the character and give the notification.
So, We can type like this :
/* Source Code from The Logic */
compare(File1,File2):-seeing(S),openandread(File1,File2),see(File1),seen,see(File2),seen,see(S).
openandread(File1,File2):-see(File1),read(X),see(File2),read(Y),(X=Y,write(X),write(' is the same as '),write(Y),nl;X\=Y,write(X),write(' is different from '),write(Y),nl),process(X,Y,File1,File2).
process(end,end,_,_).
process(_,_,File1,File2):-openandread(File1,File2).
This source code means that predicate compare must be input by name of the first file and second file. After the predicate compare receive the name of both files, the program will open and read each character from the files. After then, program will check the character. If the character is similar or identic, program will print the character and give string "as the same as" beetwen it. But if the character not similar or identic, the program will give string "is different from" beetwen it.
Step 1
Type the logic to text editor (ex. Notepad)
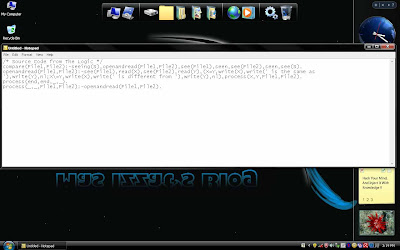
(Click the image if you can't see well)
Step 2
Save it at directory My Documents\Prolog. Save it by extention (*.pl) or (*.pro). For example named it "compare.pro".
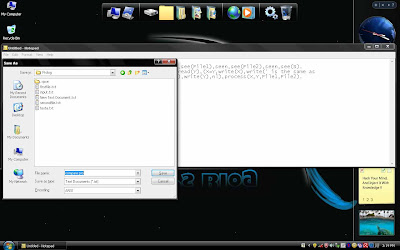
(Click the image if you can't see well)
Step 3
Make two files (Recommended by extention *.txt) at directory My Documents\Prolog. For example : named it "first.txt", "second.txt" and "output.txt".
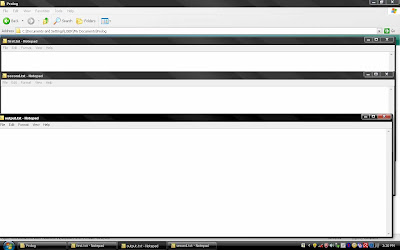
(Click the image if you can't see well)
Step 4
Start Prolog and open the source code with command consult.
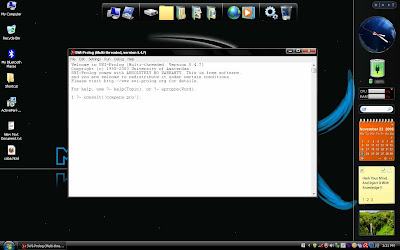
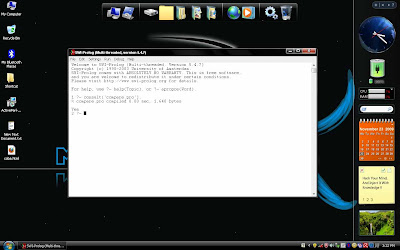
(Click the image if you can't see well)
Step 5
Now, open the first.txt and type like this :
one.
two.
three.
four.
end.
And Save it.
Note : Don't forget to type the dot mark "." at every last word and must ended by type "end".
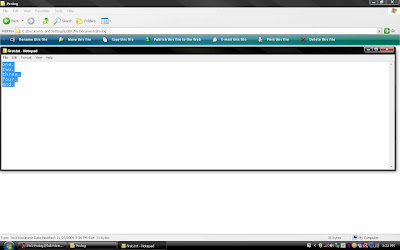
(Click the image if you can't see well)
Step 6
And then open the second.txt and type like this :
one.
second.
three.
fourth.
end.
And Save it.
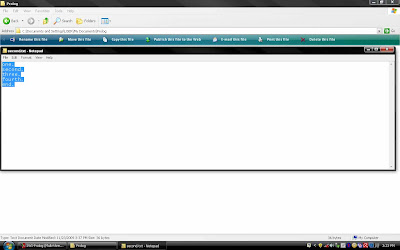
(Click the image if you can't see well)
Step 7
Now, type like this on Prolog :
compare('first.txt','second.txt','output.txt').
And hit Enter.
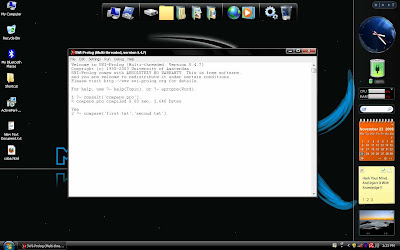
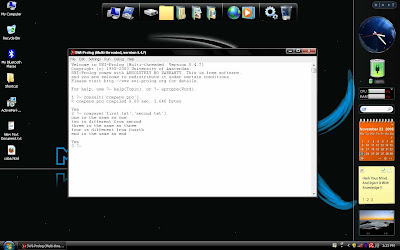
(Click the image if you can't see well)
Step 8
You can try the different word by yourself.
First, We'll show about making lower case in Prolog from User's Input. This is the source code :
/* Source Code Lower Case */
lowercase:-get0(A),process(A).
process(13).
process(A):-A=\=13,converter(A,B),put(B),makelower.
converter(A,B):-A>=65,A=<90,b>90.
This is mean if user type "lowercase", Program will ready to receive Input from user. The function of command get0() is to change one or more character to be ASCII Value. Then the ASCII value read by command process. After then, process initialize ASCII value 13 (13 means marriage return or Enter Key). If user type Enter Key, program will check the ASCII value which handled by command process before. If command proccess don't read an "Enter Key" again, Program start function converter which have function to convert ASCII value from 65 (65 is ASCII value of Upper Case A) until 90 (90 is ASCII value of Upper Case Z) by sum it by 32 (Because lower case of A is 97 in ASCII. It's same with another alphabet until Z, just sum it by 32). Then, if in process of convert, program read another character (not alphabet) Program just print it without sum the ASCII value by 32.
Step 1
Type the source code in text editor (ex. notepad).
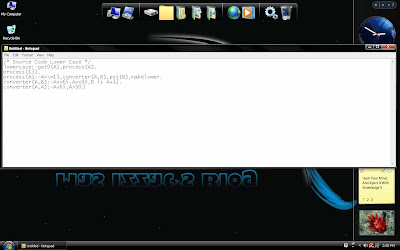
(Click the image if you can't see well)
Step 2
Save it at directory My Documents\Prolog and named it by extention (*.pl) or (*.pro). For example named it "lowercase.pro".
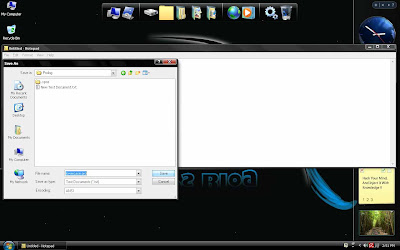
(Click the image if you can't see well)
Step 3
Open it with command consult.
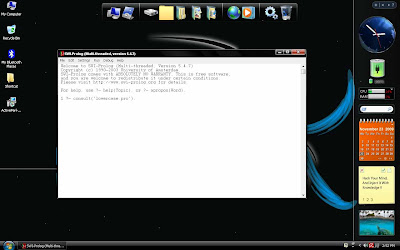
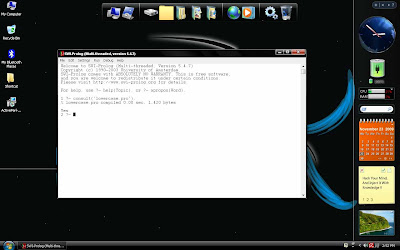
(Click the image if you can't see well)
Step 4
Now, let's check our program. Type "lowercase" (without quotes) and hit Enter.
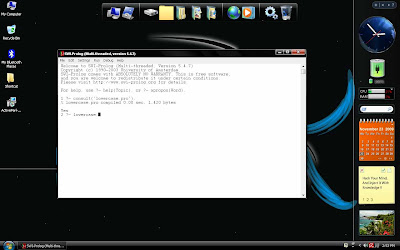
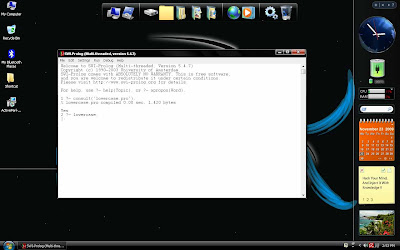
(Click the image if you can't see well)
Step 5
Try to type Anything character. For example type like this : "This is an Example 123 inCLUDing numbers and symbols +-*/@[] XYz" (Without quotes) and hit Enter
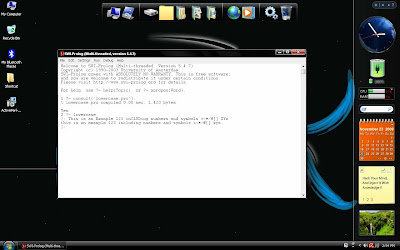
(Click the image if you can't see well)
Step 6
Let's try with different input : "ThIs IS jUSt WoRD tO Try ThE PRoGRAm !@#$%^&*()_+" (remember without quotes and hit Enter after type it)
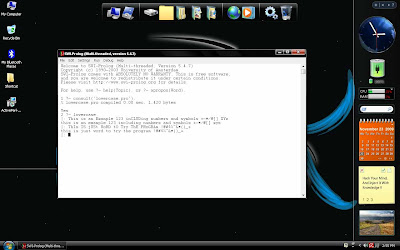
(Click the image if you can't see well)
This is the second Tutorial. Now, We will show about how to make a program in Prolog which can copy character from a file to another file.
First, let's make a logic from the program. The logic is the program must open the file and check the line per line. In each line, the program must know about the end line and then check the next line. In Prolog, There is command to Open and Write to another file. The commands are see and tell. Another commands are seeing and telling. Seeing and telling must be a variable and will normally be unbound. So, We can write the code like this :
/* Source Code Input from File */
copyterms(Infile,Outfile):-seeing(S),telling(T),see(Infile),tell(Outfile),copy_characters,seen,see(S),told,tell(T).
copy_characters:-read(N),process(N).
process(end_of_file).
process(N):-N\=end_of_file,write(N),write('.'),nl,copy_characters.
This code means that predicate copyterms must be input by file input and file output. Seeing and see in this source code have variable for input, the variable is S. Telling and tell have variable T. The precicate copy_character will read each character and the result of it will be handled by predicate process. Predicate process initialize String "end_of_file". And then, the program will check each character which handled by predicate process. If the character not a String "end_of_file", the program will print it.
Step 1
Type the source code to the text editor (Ex. Notepad)
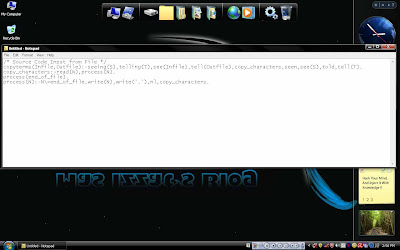
(Click the image if you can't see well)
Step 2
Save it at directory My Documents\Prolog. Save it by extention (*.pl) or (*.pro). For example named it "copyterms.pro".
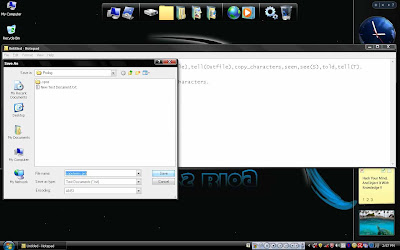
(Click the image if you can't see well)
Step 3
Make two files (Recommended by extention *.txt) at directory My Documents\Prolog. You must make 2 files. One for Input, and the another to output. For example : named it "input.txt" and "output.txt".
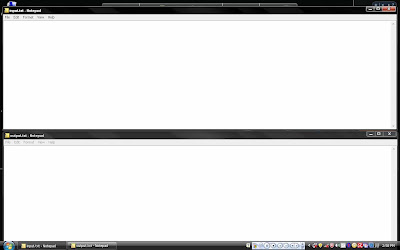
(Click the image if you can't see well)
Step 4
Start Prolog and open the source code with command consult.
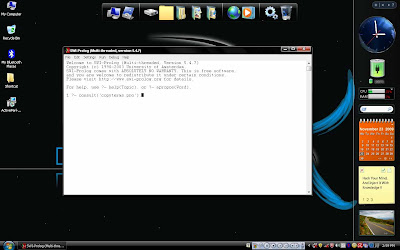
(Click the image if you can't see well)
Step 5
Now, open the input.txt and type like this :
'first term'. 'second term'.
'third term'.
fourth. 'fifth term'.
sixth.
And then save it.
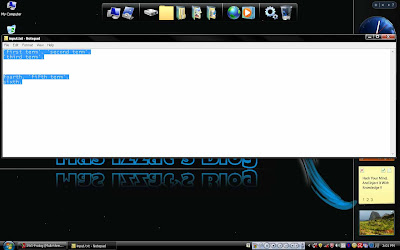
(Click the image if you can't see well)
Step 6
Type like this on Prolog : copyterms('input.txt','output.txt').
And hit Enter.
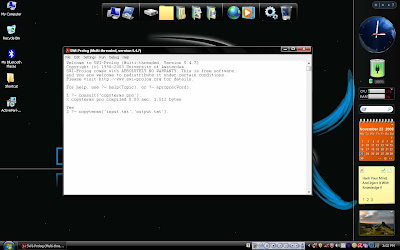
(Click the image if you can't see well)
Step 7
If Prolog show the notification "Yes", So succesfully copy the character. Now, check your output file and see the characters have been copied successfully.
Note : If your file have any blank line, the program will delete it.
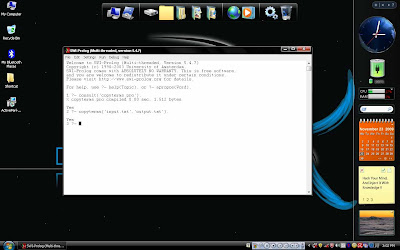
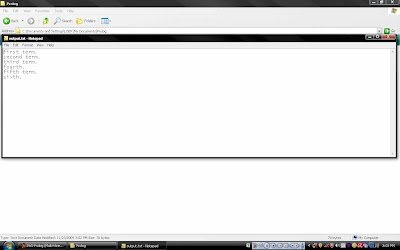
(Click the image if you can't see well)
Step 8
Let's try with different input. Type like this in your input file :
'I am'. just.
check. 'my program'.
'I hope'. this.
is.
can. compiled.
successfully.
And Save it.
Note : You must type '' mark if your input in more than one word. For example : 'I am'. And your each input must ended by stop mark ".".
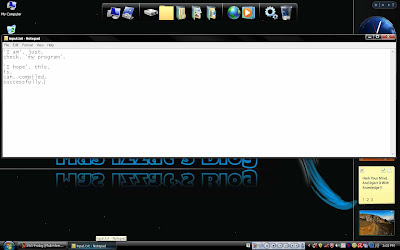
(Click the image if you can't see well)
Step 9
Type like this on Prolog again : copyterms('input.txt','output.txt'). And hit Enter.
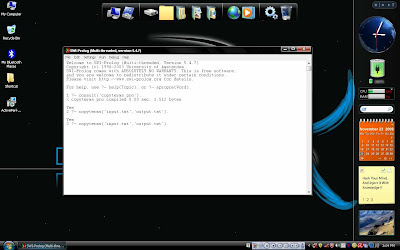
(Click the image if you can't see well)
Step 10
If Prolog show the notification "Yes", So succesfully copy the character. Now, check your output file and see the characters have been copied successfully.
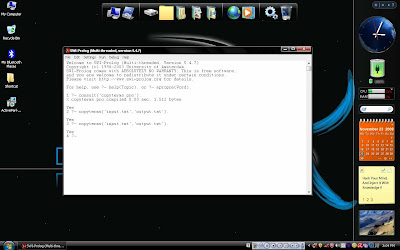
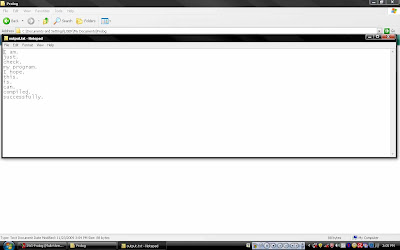
(Click the image if you can't see well)
This is the third tutorial from us. We will show about how to read one by one character from a file and convert it to ASCII value on Prolog.
Like usually, let's make the logic of program.
First, program will open a file. And then program will read one by one character. After then program will convert the character to ASCII value and the last print it on screen.
We can write the logic like this :
/* Source Code */
readfile(O):-seeing(S),see(O),readeachcharacter,readeachcharacter,readeachcharacter,readeachcharacter,readeachcharacter,readeachcharacter,readeachcharacter,readeachcharacter,readeachcharacter,readeachcharacter,seen,see(S).
readeachcharacter:-get0(A),write(A),nl.
This source code means that predicate readfile must be input by the input file name. After that program will open the file with predicate see. The predicate readeachcharacter use to convert one by one character which read by predicate readfile to be ASCII value (By predicate get0). Predicate readeachcharacter use to convert A CHARACTER from file, so We must type the predicate readeachcharacter same with the total of character will be converted.
For example, We want to convert ten character from file, So We must type predicate readeachcharacter ten times.
Step 1
Type the logic to text editor (ex. Notepad)
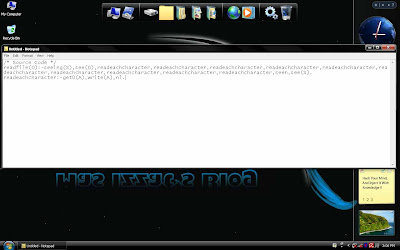
(Click the image if you can't see well)
Step 2
Save it at directory My Documents\Prolog. Save it by extention (*.pl) or (*.pro). For example named it "readfile.pro".
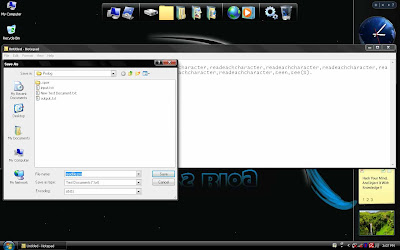
(Click the image if you can't see well)
Step 3
Make a file (Recommended by extention *.txt) at directory My Documents\Prolog. For example : named it "testa.txt".
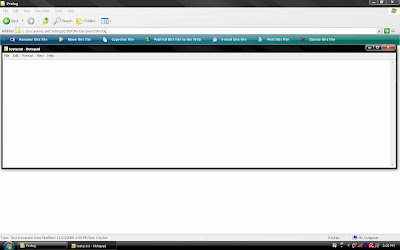.jpg)
(Click the image if you can't see well)
Step 4
Start Prolog and open the source code with command consult.
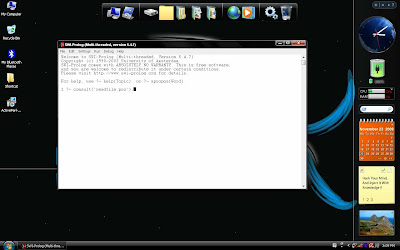
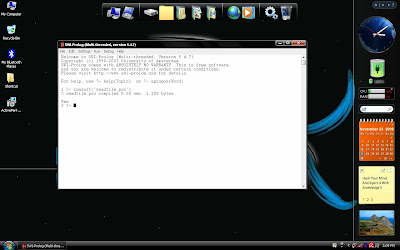
(Click the image if you can't see well)
Step 5
Now, open the input.txt and type like this :
abcde
fghij
And then save it.
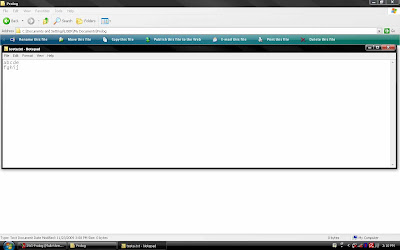
(Click the image if you can't see well)
Step 6
Type like this on Prolog : readfile('testa.txt').
And hit Enter.
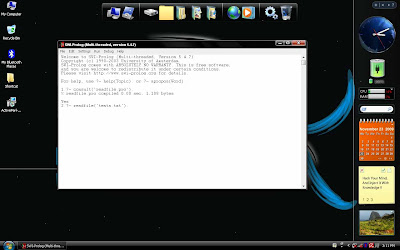
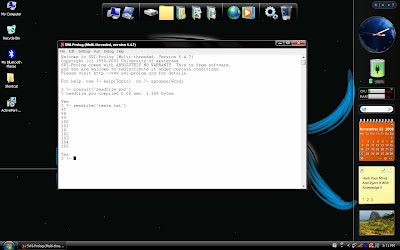
(Click the image if you can't see well)
Step 7
You can try with different word. But don't forget to change the total predicate readeachfile if you want to convert more or less character.
This is the fourth tutorial. In this tutorial, We will show about how to combine a file with another using Prolog.
Now, let's make the logic of program.
First, Program will open the first file. Then read each character in it. And then write the same charater in the output file.
Secondly, Program will open the the second file. Then read each character in it. After then write the same character in the output file.
So, We can type like this :
/* Source Code from The Logic */
combine(File1,File2,Out):-seeing(S),telling(T),tell(Out),see(File1),copycharacter,seen,see(File2),copycharacter,seen,see(S),nl,told,telling(T).
copycharacter:-read(N),process(N).
process(end).
process(N):-N\=end,write(N),nl,copycharacter.
This source code means that predicate combine must be input by name of the first file, the second file and the output file. After predicate combine receive the name of files, it will open the first file, read each character and write the same character in the output file. And then open the second file, read each character and write in the output file. On process, if character not identic with String "end", Program will copy it to the output file.
Step 1
Type the logic to text editor (ex. Notepad)
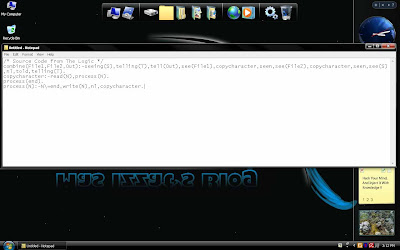
(Click the image if you can't see well)
Step 2
Save it at directory My Documents\Prolog. Save it by extention (*.pl) or (*.pro). For example named it "combine.pro".
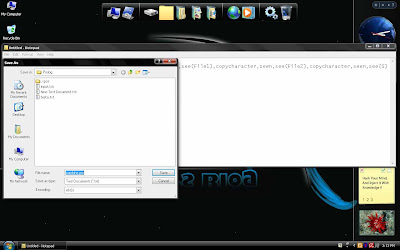
(Click the image if you can't see well)
Step 3
Make three files (Recommended by extention *.txt) at directory My Documents\Prolog. For example : named it "firstfile.txt", "secondfile.txt" and "output.txt".
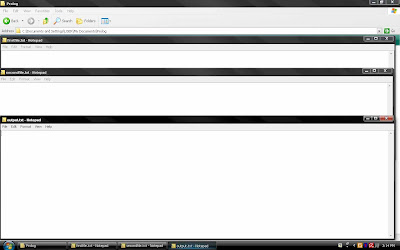
(Click the image if you can't see well)
Step 4
Start Prolog and open the source code with command consult.
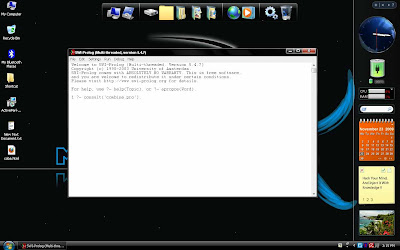
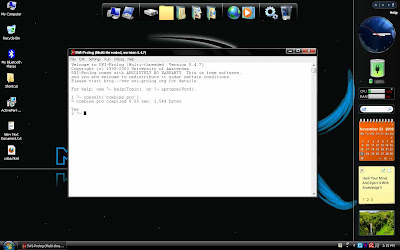
(Click the image if you can't see well)
Step 5
Now, open the firstfile.txt and type like this :
just.
try.
the.
program.
end.
And Save it.
Note : Don't forget to type the dot mark "." at every last word and must ended by type "end".
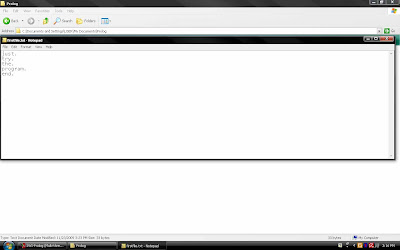
(Click the image if you can't see well)
Step 6
And then open the secondfile.txt and type like this :
but.
do.
not.
forget.
to.
type.
the.
dot.
mark.
and.
must.
ended.
by.
string-end.
end.
And Save it.
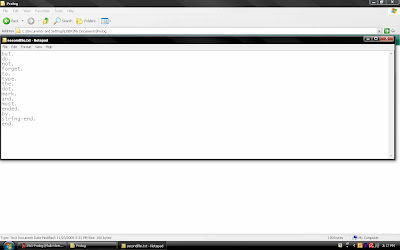
(Click the image if you can't see well)
Step 7
Now, type like this on Prolog :
combine('firstfile.txt','secondfile.txt','output.txt').
And hit Enter
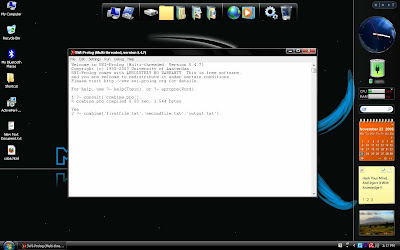
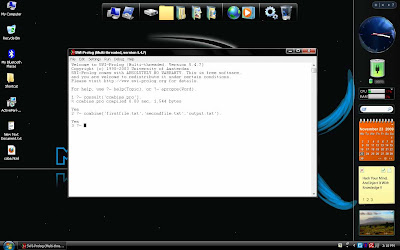
(Click the image if you can't see well)
Step 8
Open the output file to check the character.
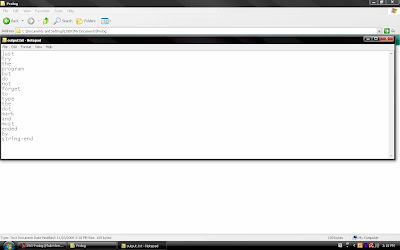
(Click the image if you can't see well)
Step 9
You can try the different word by yourself.
This the last tutorial from us now. This tutorial is about how to read each character from 2 files and check the character is the same character or not.
Okay, let's make the logic.
First, program will open the first file and the second file and then read the each character.
Secondly, check the character from first file and second file. If the character from the first file and the second file is identic, the program will print the character and give the notification.
So, We can type like this :
/* Source Code from The Logic */
compare(File1,File2):-seeing(S),openandread(File1,File2),see(File1),seen,see(File2),seen,see(S).
openandread(File1,File2):-see(File1),read(X),see(File2),read(Y),(X=Y,write(X),write(' is the same as '),write(Y),nl;X\=Y,write(X),write(' is different from '),write(Y),nl),process(X,Y,File1,File2).
process(end,end,_,_).
process(_,_,File1,File2):-openandread(File1,File2).
This source code means that predicate compare must be input by name of the first file and second file. After the predicate compare receive the name of both files, the program will open and read each character from the files. After then, program will check the character. If the character is similar or identic, program will print the character and give string "as the same as" beetwen it. But if the character not similar or identic, the program will give string "is different from" beetwen it.
Step 1
Type the logic to text editor (ex. Notepad)
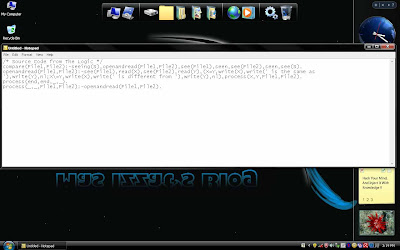
(Click the image if you can't see well)
Step 2
Save it at directory My Documents\Prolog. Save it by extention (*.pl) or (*.pro). For example named it "compare.pro".
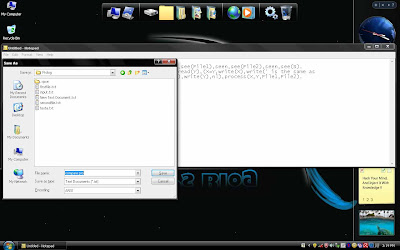
(Click the image if you can't see well)
Step 3
Make two files (Recommended by extention *.txt) at directory My Documents\Prolog. For example : named it "first.txt", "second.txt" and "output.txt".
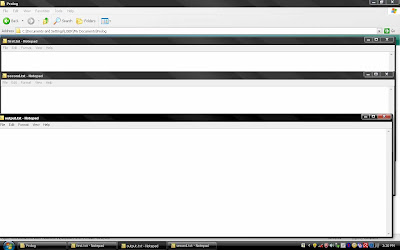
(Click the image if you can't see well)
Step 4
Start Prolog and open the source code with command consult.
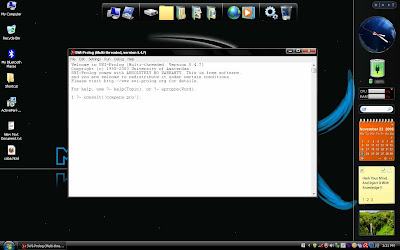
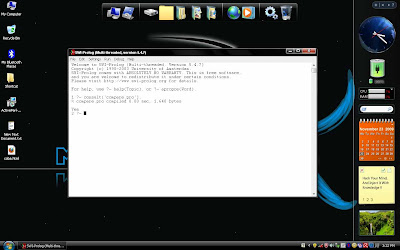
(Click the image if you can't see well)
Step 5
Now, open the first.txt and type like this :
one.
two.
three.
four.
end.
And Save it.
Note : Don't forget to type the dot mark "." at every last word and must ended by type "end".
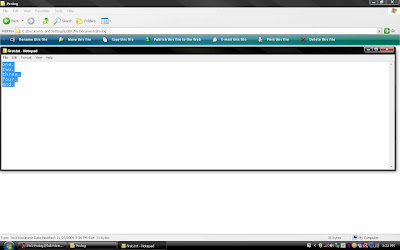
(Click the image if you can't see well)
Step 6
And then open the second.txt and type like this :
one.
second.
three.
fourth.
end.
And Save it.
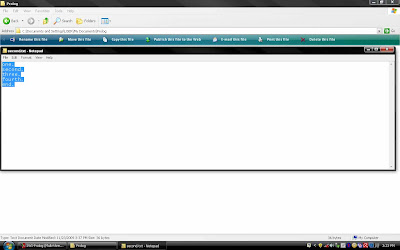
(Click the image if you can't see well)
Step 7
Now, type like this on Prolog :
compare('first.txt','second.txt','output.txt').
And hit Enter.
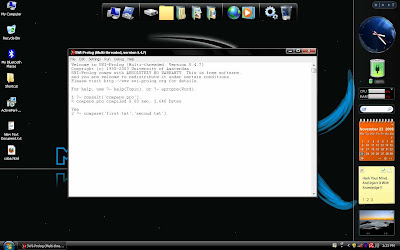
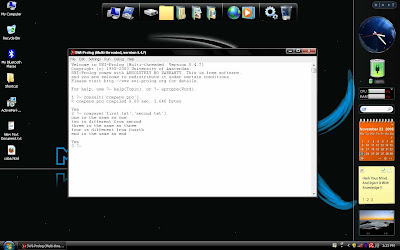
(Click the image if you can't see well)
Step 8
You can try the different word by yourself.
0 comments:
Post a Comment