In this post, We make two tutorial.
First, We will show about how to program Prolog which will make output the animal chases another animal or not. This is the source code :
dog(fido). large(fido).
cat(mary). large(mary).
dog(rover). small(rover).
cat(jane). small(jane).
dog(tom). small(tom).
cat(harry).
dog(fred). large(fred).
cat(henry). large(henry).
cat(bill).
cat(steve). large(steve).
large(jim).
large(mike).
large_dog(X):- dog(X),large(X).
small_animal(A):- dog(A),small(A).
small_animal(B):- cat(B),small(B).
chases(X,Y):-
large_dog(X),small_animal(Y),
write(X),write(' chases '),write(Y),nl.
It means that if user type chases(animal1,animal2) which animal1 and aimal2 is member of source, Prolog will check "Is animal1 a large dog and animal2 a small dog OR small cat OR not ?". If It's true, Prolog will make output animal1 chases animal2. And if It's false, Prolog will make output No.
But, what about if We want to use operator ?. We use this source :
?-op(150,xf,is_large).
?-op(150,xf,is_small).
?-op(150,xf,isa_large_dog).
?-op(150,xf,isa_small_animal).
?-op(150,xfy,chases).
fido isa_dog. fido is_large.
mary isa_cat. mary is_large.
rover isa_dog. rover is_small.
jane isa_cat. jane is_small.
tom isa_dog. tom is_small.
harry isa_cat.
fred isa_dog. fred is_large.
henry isa_cat. henry is_large.
bill isa_cat.
steve isa_cat. steve is_large.
jim is_large.
mike is_large.
X isa_large_dog:- X isa_dog,X is_large.
A isa_small_animal:- A isa_dog,A is_small.
B isa_small_animal:- B isa_cat,B is_small.
X chases Y:- X isa_large_dog,Y isa_small_animal,write(X),write(' chases '),write(Y),nl.
Note :"op" is a predicate at the system prompt. 150 is the 'operator precedence', which is an integer from 0 upwards. The range of numbers used depends on the particular implementation. The lower the number, the higher the precedence. Operator precedence values are used to determine the order in which operators will be applied when more than one is used in a term. The most important practical use of this is for operators used for arithmetic, as will be explained later. In most other cases it will suffice to use an arbitrary value such as 150. The second argument should normally be one of the following three atoms: xfy meaning that the predicate is binary and is to be converted to an infix operator fy meaning that the predicate is unary and is to be converted to an prefix operator xf meaning that the predicate is unary and is to be converted to a postfix operator. The third argument specifies the name of the predicate that is to be converted to an operator.
Step 1Type the source in the text editor (ex. notepad). This is the code of first program and second program (using operator)
Save it by extention (*.pl) or (*.pro). For example, We named it animal2.pro for the first program, and animal3.pro for the second program.
Note : Must be saved in directory My Documents\Prolog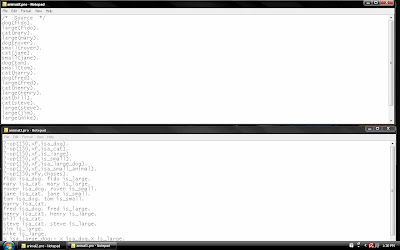
(Click the image if you can't see well)
Step 2Now, open Prolog and open the file with command consult.
Don't worry about notification from Prolog.
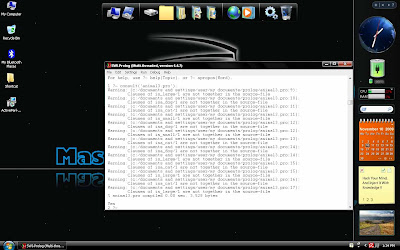
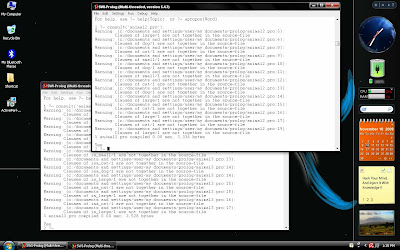
(Click the image if you can't see well)
Step 3Okay, let's check our program. For example, type chases(fido,tom). Or you can type "fido chases tom". (without quotes)
Prolog make output "fido chases tom". It's because fido is large dog and tom is small dog. So fido can chases tom.
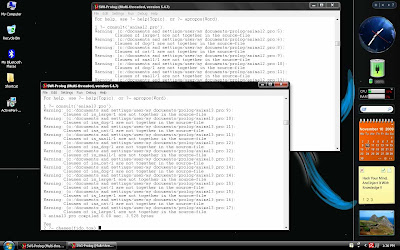
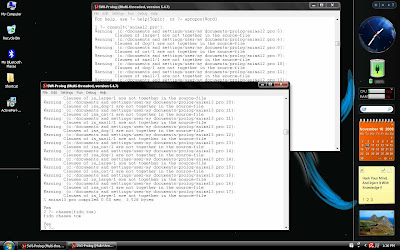
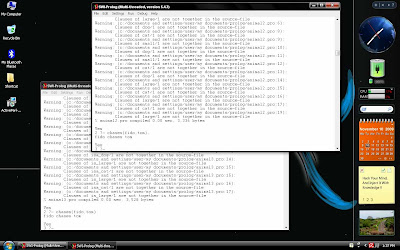
(Click the image if you can't see well)
Step 4Let's check again with another name.
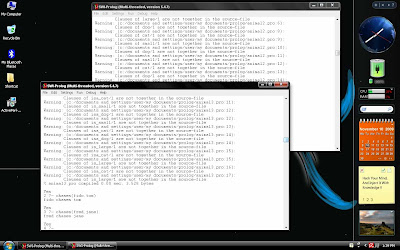
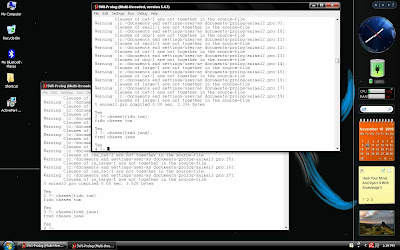
(Click the image if you can't see well)
Now, this is the second tutorial. It's about how to program Prolog which can define and test a predicate which takes two arguments, both numbers, and calculates and outputs the following values: (a) their average, (b) the square root of their product and (c) the larger of (a) and (b).
The logic of the program is if user type number1 and number2, Prolog will calculate average, square root of their product, and the larger of average and square root of both numbers's product.
Step 1First, make logic which Prolog can make output average of two number. Average from two number is number1 + number2 and then divide it with 2. The logic is like this "A is (X+Y)/2." A is variable which handle the result from (X+Y)/2. X and Y are number1 and number2.
Step 2Make logic which Prolog can make output square root of both number's product. The product of two numbers is number1 * number2 or can type like this : "B is (X*Y)". B is variable which handle the result from (X*Y). X and Y is number1 and number2. And then, make square root from the result of (X*Y). The logic is like this : "C is sqrt(B)". B is variable which handle the result from (X*Y) and C is variable which handle the result from square root (X*Y).
Step 3Make logic which Prolog can make output the larger between average and square root of both numbers's product. The logic is like this : A<C,write(C),write(' is larger than '),write(A),nl;A>C,write(A),write(' is larger than '),write(C),nl.
It's means that IF A less than C then print C and give string "is larger than" and the last print A OR (";" means OR) IF A bigger than C then print A and give string "is larger than" and the last print C.
Step 4Okay, let's make the source code for our program. Type like this at text editor :
number(X,Y):-A is (X+Y)/2,nl,write('the average is '),write(A),nl,nl,B is (X*Y),C is sqrt(B),write('the squareroot from the product of numbers is '),write(C),nl,nl,(A<C,write(C),write(' is larger than '),write(A),nl;A>C,write(A),write(' is larger than '),write(C),nl).
This code consist of average logic, square root of both numbers's product logic, and larger output logic.
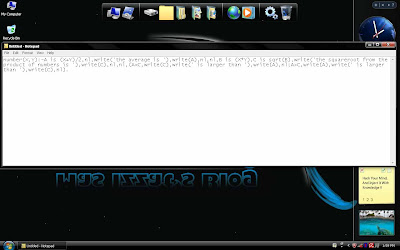.jpg)
(Click the image if you can't see well)
Step 5Now, save it by extention (*.pl) or (*.pro). For example, We named it number.pro.
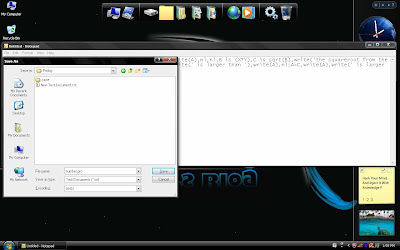.jpg)
(Click the image if you can't see well)
Step 6Now, open Prolog and open the file with command consult.
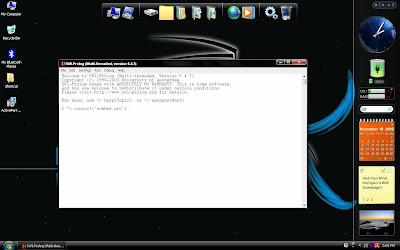
(Click the image if can't see well)
Step 7This is time to check our program. First, try it by typing : number(1,2).
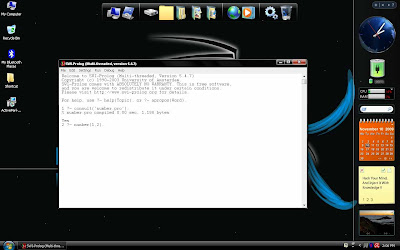
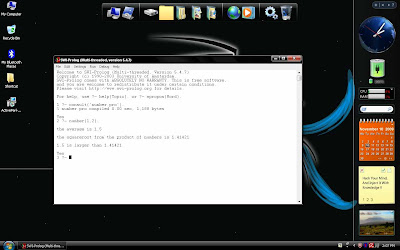.jpg)
(Click the image if can't see well)
Then, try it by typing : number(5,3).
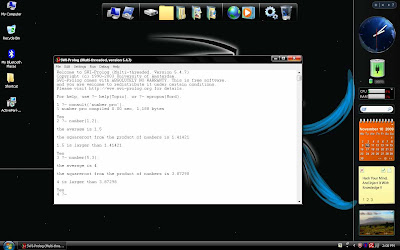.jpg)
(Click the image if you can't see well)
Read more...